chris1 Code is Art
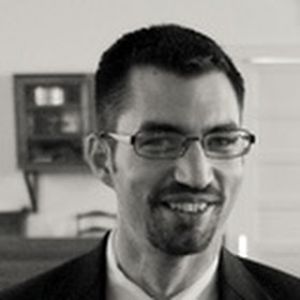 Posts: 3862 Reg: Aug 10, 2012 Austin, TX
|
06/24/13 12:37 AM
(10 years ago)
|
|
chris1 Code is Art
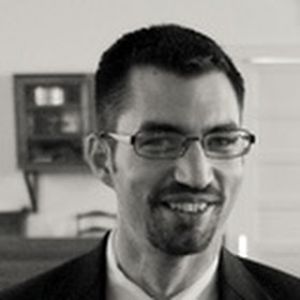 Posts: 3862 Reg: Aug 10, 2012 Austin, TX | 06/24/13 12:42 AM (10 years ago) |
|
Joe Sprott Code is Art
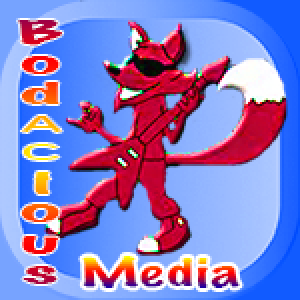 Posts: 414 Reg: Aug 20, 2011 Melbourne, FL | 06/24/13 12:45 AM (10 years ago) |
|
chris1 Code is Art
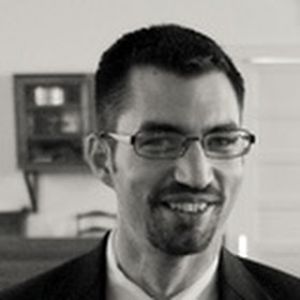 Posts: 3862 Reg: Aug 10, 2012 Austin, TX | 06/24/13 12:47 AM (10 years ago) |
|
Joe Sprott Code is Art
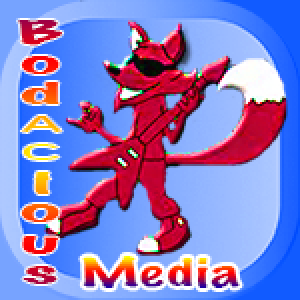 Posts: 414 Reg: Aug 20, 2011 Melbourne, FL | 06/24/13 12:53 AM (10 years ago) |
|
chris1 Code is Art
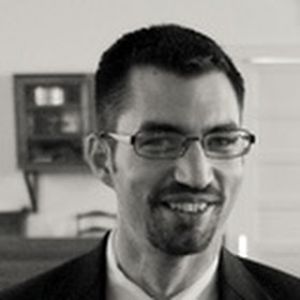 Posts: 3862 Reg: Aug 10, 2012 Austin, TX | 06/24/13 12:54 AM (10 years ago) |
|
Kittsy buzztouch Evangelist
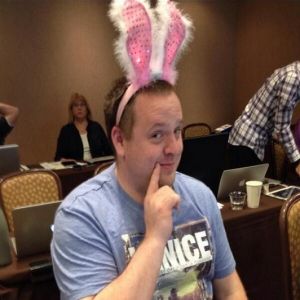 Posts: 2251 Reg: Feb 22, 2012 Liverpool | 06/24/13 12:55 AM (10 years ago) |
|
mrDavid BTMods.com
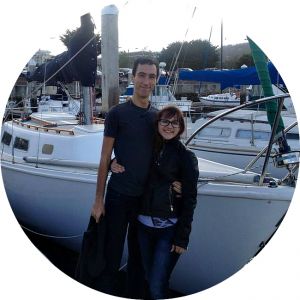 Posts: 3936 Reg: May 21, 2011 San Diego, CA | 06/24/13 02:33 AM (10 years ago) |
|
SmugWimp Smugger than thou...
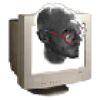 Posts: 6316 Reg: Nov 07, 2012 Tamuning, GU | 06/24/13 04:17 AM (10 years ago) |
|
Jake Chasan Veteran developer
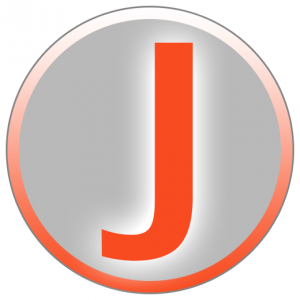 Posts: 1685 Reg: May 13, 2011 location unknow... | 06/24/13 07:53 AM (10 years ago) |
|
PSMDanny Apple Fan
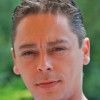 Posts: 1166 Reg: Dec 09, 2011 Heerlen | 06/24/13 11:13 AM (10 years ago) |
|
SheriDee Code is Art
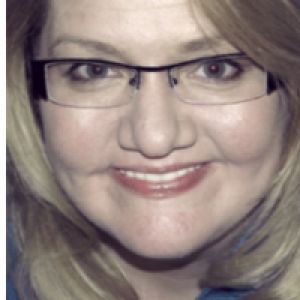 Posts: 1094 Reg: Sep 23, 2011 location unknow... | 06/24/13 11:49 AM (10 years ago) |
|
WebNevees Code is Art
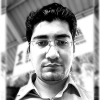 Posts: 206 Reg: Oct 28, 2012 KL | 06/24/13 12:04 PM (10 years ago) |
|
David @ buzztouch buzztouch Evangelist
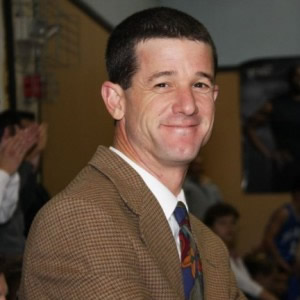 Posts: 6866 Reg: Jan 01, 2010 Monterey, CA | 06/25/13 01:11 AM (10 years ago) |
|
mysps Code is Art
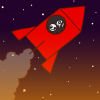 Posts: 2082 Reg: May 14, 2011 Palma | 06/25/13 09:58 AM (10 years ago) |
|
Login + Screen Name Required to Post
Login to participate
so you can start
earning points.
Once you're logged in (and have a screen name entered in your profile), you can subscribe to topics, follow users, and start learning how to make apps
like the pros.
|