GoNorthWest buzztouch Evangelist
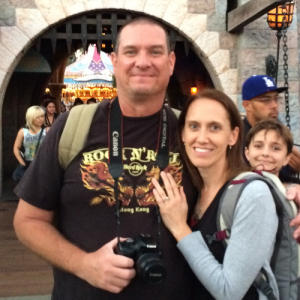 Posts: 8197 Reg: Jun 24, 2011 Oro Valley, AZ
|
01/22/13 12:37 PM
(11 years ago)
|
|
PSMDanny Apple Fan
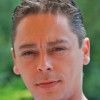 Posts: 1166 Reg: Dec 09, 2011 Heerlen | 01/22/13 12:51 PM (11 years ago) |
|
Paul Rogers Android Fan
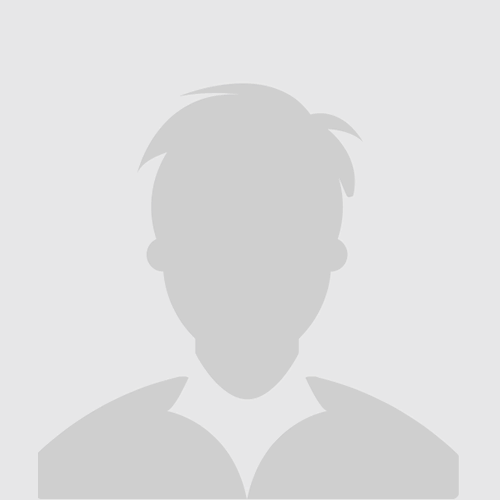 Posts: 2524 Reg: May 30, 2011 UK | 01/22/13 01:12 PM (11 years ago) |
|
LA Aspiring developer
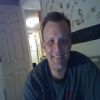 Posts: 3278 Reg: Aug 16, 2012 Jerseyville, IL | 01/22/13 01:13 PM (11 years ago) |
|
GoNorthWest buzztouch Evangelist
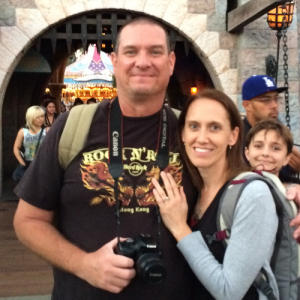 Posts: 8197 Reg: Jun 24, 2011 Oro Valley, AZ | 01/22/13 01:15 PM (11 years ago) |
|
SmugWimp Smugger than thou...
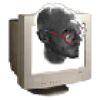 Posts: 6316 Reg: Nov 07, 2012 Tamuning, GU | 01/22/13 02:11 PM (11 years ago) |
|
trailman Aspiring developer
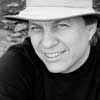 Posts: 280 Reg: Dec 10, 2010 Sedona, az6,550 | 01/22/13 02:23 PM (11 years ago) |
|
GoNorthWest buzztouch Evangelist
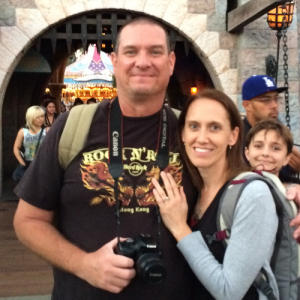 Posts: 8197 Reg: Jun 24, 2011 Oro Valley, AZ | 01/22/13 02:37 PM (11 years ago) |
|
Mr stuck Android Fan
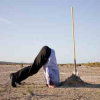 Posts: 974 Reg: Apr 09, 2012 Fife, Scotland15,740  | 01/22/13 04:22 PM (11 years ago) |
|
newsight Lost but trying
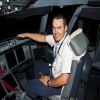 Posts: 14 Reg: Jan 14, 2013 Sydney3,540 | 01/23/13 03:07 AM (11 years ago) |
|
james007 Veteran developer
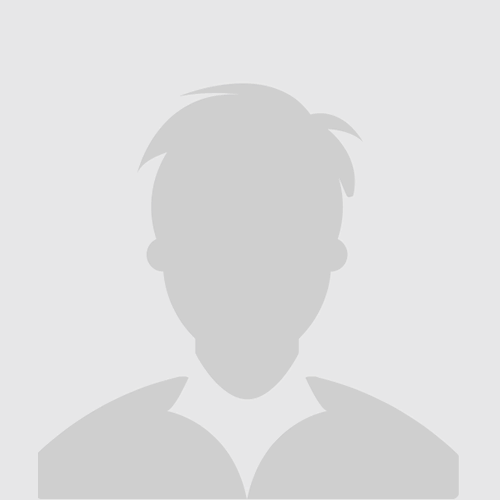 Posts: 2 Reg: Sep 26, 2014 Sunnyvale20 | 09/26/14 06:15 AM (9 years ago) |
|
sarahk Code is Art
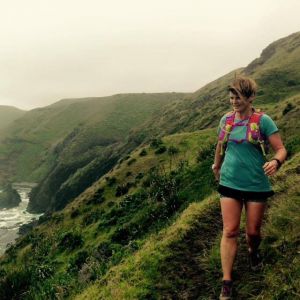 Posts: 159 Reg: Jul 16, 2014 Auckland | 03/11/16 02:19 AM (8 years ago) |
|
SmugWimp Smugger than thou...
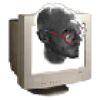 Posts: 6316 Reg: Nov 07, 2012 Tamuning, GU | 03/11/16 03:05 AM (8 years ago) |
|
GoNorthWest buzztouch Evangelist
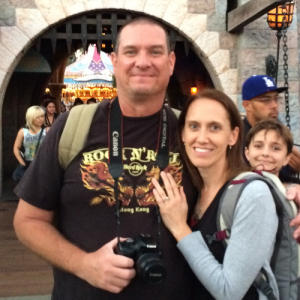 Posts: 8197 Reg: Jun 24, 2011 Oro Valley, AZ | 03/11/16 07:28 AM (8 years ago) |
|
Login + Screen Name Required to Post
Login to participate
so you can start
earning points.
Once you're logged in (and have a screen name entered in your profile), you can subscribe to topics, follow users, and start learning how to make apps
like the pros.
|